Introduction
Java provides multiple ways to write or print a line to a file, making it a versatile language for handling file I/O operations. Whether you are working on a small script or a large-scale application, understanding how to efficiently print output to a file is essential. This guide will cover various methods for writing lines to a file in Java, ensuring you have a clear and practical understanding of each approach.
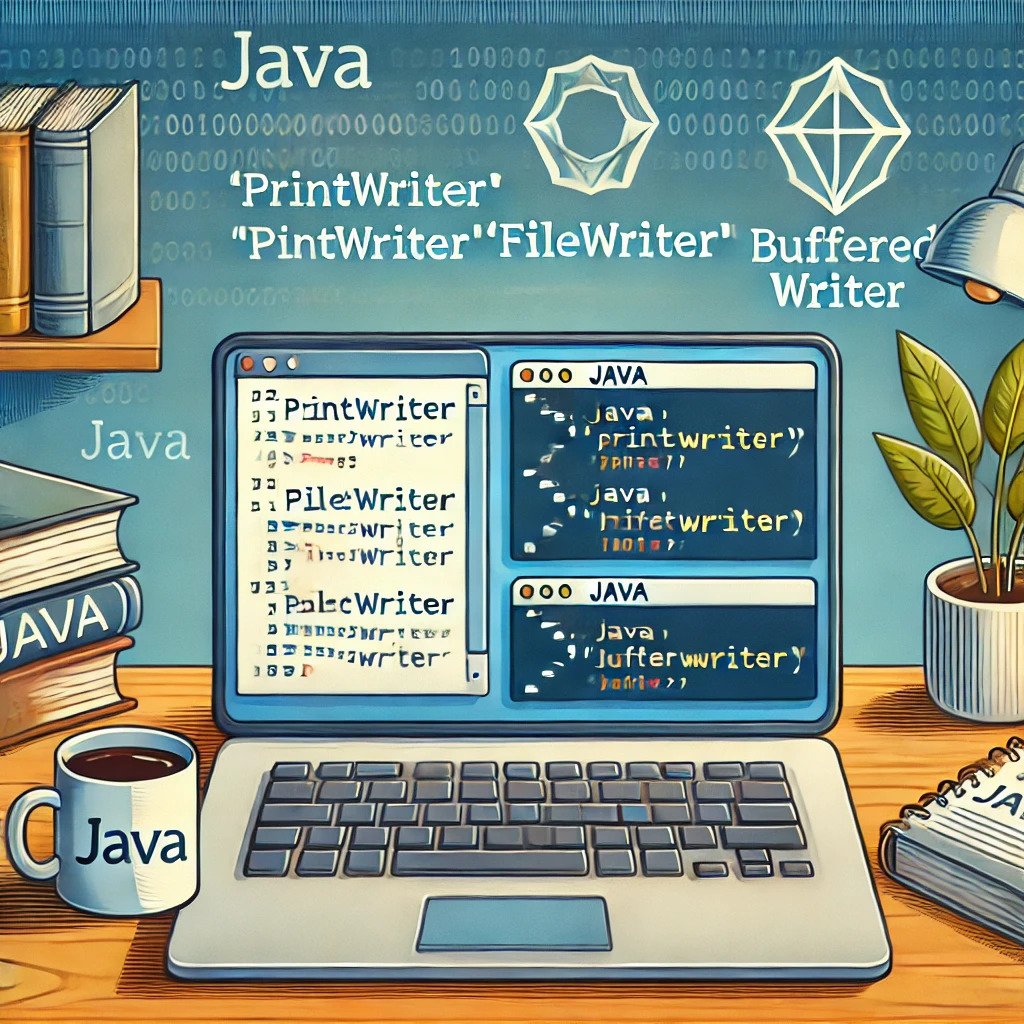
Why Write to a File in Java?
Writing to a file is a common requirement in software development. Some typical use cases include:
- Logging: Storing log data for debugging and monitoring.
- Data Storage: Saving user inputs or processed data.
- File-Based Communication: Exchanging data between different systems.
Printing a Line to a File in Java
1. Using PrintWriter
The PrintWriter class provides a simple and efficient way to write data to a file. It supports formatted text and automatic line flushing.
Example:
import java.io.*;
public class PrintWriterExample {
public static void main(String[] args) {
try (PrintWriter writer = new PrintWriter(“output.txt”)) {
writer.println(“Hello, world!”);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Advantages:
- Easy to use
- Supports formatted output
- Automatically flushes output when writing a line
2. Using FileWriter
The FileWriter class is another way to write data to a file in Java. It provides control over whether to append or overwrite a file.
Example:
import java.io.*;
public class FileWriterExample {
public static void main(String[] args) {
try (FileWriter writer = new FileWriter(“output.txt”)) {
writer.write(“Hello, world!\n”);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Advantages:
- Supports character encoding
- Can append data by setting append to true
3. Using BufferedWriter
For writing large amounts of text efficiently, BufferedWriter is an excellent choice. It minimizes I/O operations by buffering output data.
Example:
import java.io.*;
public class BufferedWriterExample {
public static void main(String[] args) {
try (BufferedWriter writer = new BufferedWriter(new FileWriter(“output.txt”))) {
writer.write(“Hello, world!”);
writer.newLine();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Advantages:
- Improves performance by reducing direct file writes
- Supports efficient line breaks
4. Using Files.write()
The Files.write() method is part of Java NIO and is a modern approach for writing to files.
Example:
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.Collections;
public class FilesWriteExample {
public static void main(String[] args) {
try {
Files.write(Paths.get(“output.txt”), Collections.singletonList(“Hello, world!”));
} catch (IOException e) {
e.printStackTrace();
}
}
}
Advantages:
- Compact and simple
- Uses modern Java features
5. Using FileOutputStream
For writing binary or raw data, FileOutputStream is useful.
Example:
import java.io.*;
public class FileOutputStreamExample {
public static void main(String[] args) {
try (FileOutputStream fos = new FileOutputStream(“output.txt”)) {
fos.write(“Hello, world!”.getBytes());
} catch (IOException e) {
e.printStackTrace();
}
}
}
Advantages:
- Supports writing both text and binary data
Best Practices for Writing to a File in Java
- Always close the file writer: Use try-with-resources to automatically close file writers.
- Handle exceptions properly: Use try-catch blocks to manage errors.
- Use buffering for large files: BufferedWriter improves performance.
- Specify character encoding: Always define encoding to avoid compatibility issues.
- Optimize performance: Minimize I/O operations by writing efficiently.
READ ABOUT:Java Keyboard Scanner: An All-Inclusive Manual for Novices
FAQs
1. How do I append a line to an existing file in Java?
You can append data using FileWriter by setting append to true:
new FileWriter(“output.txt”, true)
2. Which method is best for writing large files in Java?
Using BufferedWriter is the best approach for handling large files efficiently.
3. How can I ensure my file writes are thread-safe?
Use synchronized blocks or java.nio.channels.FileLock for thread safety.
4. What is the difference between PrintWriter and BufferedWriter?
PrintWriter provides formatted printing, while BufferedWriter improves performance by reducing direct I/O operations.
5. Can I write to a file without overwriting existing content?
Yes, by using FileWriter with append mode:
new FileWriter(“output.txt”, true)
Conclusion
Printing a line to a file in Java is a fundamental operation, and various methods provide flexibility depending on the use case. Whether you need simple text output, high-performance writing, or binary file handling, Java has robust tools to meet your needs. By following best practices and choosing the right method, you can efficiently manage file I/O operations in your applications.